Construction of Omni-directional Robocup Robot using LEGO Click to download lego_nxt_robocup.pdf file (2.4MBytes) detailing the construction details using Lego NXT and with omniwheels or Holonomic wheels. Click to download Lego NXT-G MyBlock and demo codes holonomicnxt.zip which is about 3MBytes. These codes provide omni-directional movement using omniwheels. Programs in C written for BRICX NXC compiler is listed below. //======================================= // This demo program is to demonstrate how to move // a robot fitted with 3 Holonomic wheels to move // in a specified direction. // Details on the Hoplonomic wheels and robot // constructions can be found at // http://www.holonomicwheel.com // Please feel free to use this program. // I do not wish to copyright this program // so that others can enjoy and improve on it. // Thank you. // //==================================== // This routine is to get an angle using the NXT // controller buttons for demo use only. int GetAngle (void) { int angle; TextOut(0, LCD_LINE1, "Right = increase"); TextOut(0, LCD_LINE2, "Left = decrease"); TextOut(0, LCD_LINE4, "Angle = "); angle=0; do { if (ButtonPressed(BTNRIGHT, true)) angle+=2; if (ButtonPressed(BTNLEFT, true)) angle-=2; TextOut(50, LCD_LINE4, " "); // clears displayed number NumOut(50, LCD_LINE4, angle); Wait(300); } while (!ButtonPressed(BTNCENTER, true)) return angle; }
// ------------------------------- // Works on 3 Holonomic wheels // using 3 NXT motors at 60 degrees. void Holonomic(int degree, int power) { int angle, powerA, powerB, powerC, max; powerA=Cos(degree); angle=degree-30; powerB=Sin(angle); angle=degree+30; powerC=-Sin(angle); max=abs(powerA); if (max<abs(powerB)) max=abs(powerB); if (max<abs(powerC)) max=abs(powerC); powerA=powerA*power/max; powerB=powerB*power/max; powerC=powerC*power/max; OnFwd(OUT_A,powerA); OnFwd(OUT_B,powerB); OnFwd(OUT_C,powerC); }
task main() { int angle; angle=GetAngle(); Holonomic(angle,100); Wait(3000); } Construction using Lego and Holonomic Wheels gives a very unique challenge because the Lego NXT motors are quite big and there is a restriction that the robot must be within 22cm diameter. Normal construction is to place the motor vertically and thus will have a high center of gravity and may not be as stable. We will try to describe in as detail as possible with numerous photos and from different angles so that you can construct your own. This robot that took us many refinements has a low center of gravity and within 22cm. You may add a metal armour plate and secure it with cable ties or wires. Since Lego pieces may break off under severe impact, I suggest that you use adhesive tapes to secure critical pieces together Meanwhile the program code using Lego NXT-G is available below this image. 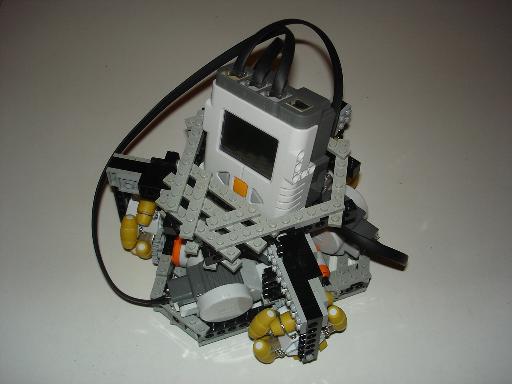
SOFTWARE Lego NXT-G The MyBlock programs for Holonomic functions to operate the robot. Those files in MyBlock folder in the zip file must be copied to MyBlock folder of the PC installed with Lego NXT-G. To access this folder, run Lego NXT-G, open any existing program or create new, then click on menu Edit then "Manage Custom Palette". You can then copy all the programs in MyBlock folder of the zip file to the MyBlock folder in "Manage Custom Palette".
There are 4 MyBlock functions that you can use for holonomic movements and several other functions used by holonomic functions and you can use those other functions too. All the files in MyBlock folder must be copied over in order for it to work. The other demo programs can be copies any where except into the MyBlock folder: HolonomicWheel.rbt This program allows you to set a direction in degrees for the robot to move. The input angle can be any degree including negative numbers. You can also set the power level from 1 to 100. with 1 the lowest power and 100 being the highest power. The exception is 0 being reserved for maximum power equivalent to 100. HoloMove.rbt This program contains allows you to specify input power to the 3 motors from values -100 to 100. The negative values make the robot turn anti-clockwise and the positive values make it turn clockwise. A power value of 0 will stop the motor. A Duration input is in seconds. The function will exit when the duration time is up and the motors will will brake. If a Duration value of 0 is used (default), the function will exit immediately but will not stop the motor.
HoloStop.rbt This program brakes all 3 motors. You can also use standard Lego stop function to stop the motors too. This function is provided for convenience instead of having to put in 3 icons to stop the 3 motors. HoloTurn.rbt This function turns the robot a specified angle. This is useful to re-align a robot if it is out of position. PowerCorrection.rbt This function is provided because under low power, the amount of motor rotation rotation is not proportional to the power level applied to the motor. For example, by specifying power level 10, the actual physical power od the motor may be at 5% of full power at 100. PowerCorrection corrects that level from 10 to 19, 20 to 28, 90 to 91, 100 remains as 100 so that it behaves closer to actual expected level. This power correction may need to be changed to cater for different ground friction or motor behaviour. Just open this block and edit the divisor. 10000xCos.rbt 10000xSin.rbt _CosAcuteAngle.rbt _SinAcuteAngle.rbt The above sin(x) and cos(x) functions are provided to compute for the angle x in degrees. X can be any angle including negative number. The return values will be from -10000 to 10000. This is because NXT-G can only operate on integer values and cannot support floating point numbers so if it were to return values like 0.5, 0.707, etc then as integer, it will always return as 0. Since NXT-G is a 4 byte integer and can support values from -2billion to 2billion, we can afford to multiple the result by 10000 to retain accuracy. The sin() and cos() functions are derived from binomial expansion. The functions _CosAcuteAngle and _SinAcuteAngle are used internally by the sin() and cos() functions. Acute.rbt This program returns an acute angle and the quadrant the angle is in. The quadrant numbers are from 1 to 4, 1 being from 0 to 90 degrees, 2 from 90 to 180 degrees etc. abs(x).rbt AbsMax.rbt Mod.rbt These functions are used by the sin() and cos() functions but you can also use them independently. They can be useful in your other programs. Please feel free to use those programs and even to modify them. Note that sometimes when these MyBlock functions may open as a broken MyBlock. This problem exists in NXT-G version 1.0 and 1.1 too and I think it is a bug in NXT-G. Normally, I would close other NXT-G programs that are open and retry or to exit and come back on again. The problem normally goes away. |